[ home ] [ math / cs / ai / phy / as / chem / bio / geo ] [ civ / aero / mech / ee / hdl / os / dev / web / app / sys / net / sec ] [ med / fin / psy / soc / his / lit / lin / phi / arch ] [ off / vg / jp / 2hu / tc / ts / adv / hr / meta / tex ] [ chat ] [ wiki ]
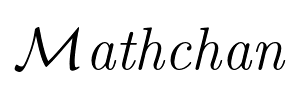
/os/ - Operating Systems Catalog
25 Dec 2021 | Mathchan is launched into public |

Sort by:
Image size:

R: 9 / I: 6
Consider a function that contains an infinite loop:
Calling the function
Now consider another function with an infinite loop:
Calling the function
What if we were to consecutively call
By default, every program is single-threaded and the only thread running is the one executing the
Once threads are created they are marked as , the scheduler will grant them the opportunity to execute at some point. The
The complete code:
Compiling the code above with
POSIX Semaphores
What is threading?Consider a function that contains an infinite loop:
void* a(void* data) { for ( ; ; ) { printf("a"); } return NULL; }
Calling the function
a();would cause the program to print "a" forever.
Now consider another function with an infinite loop:
void* b(void* data) { for ( ; ; ) { printf("b"); } return NULL; }
Calling the function
b();, it would cause the program to print "b" forever.
What if we were to consecutively call
a(); b();? Well, since
a()contains an infinite loop, the call to
b()is never going to happen, so all we're going to get is "aaaaaaaaaaaaaaaa". Similarly, if we were to call
b(); a();all we're going to get is "bbbbbbbbbbbbbbbbb". Is there a way to make the program print a little bit of "a" and a little bit of "b" (i.e. "aaaabbbbbbbaaabbbb") without changing the code (i.e. with infinite loops)? This is what threads are about. By creating threads, we make it possible for multiple infinite loops to execute concurrently. Note that there is a slight difference between concurrency and parallelism. Parallel execution means two tasks are running completely simultaneously. Concurrent execution means two tasks are switching turns: task 1 is executing, then task 2, then task 1 again... If context switching is happening fast enough (the OS scheduler is the one deciding this), it would appear as if though they were running in parallel. Most synchronization techniques work the same for both so we'll make no distinction.
By default, every program is single-threaded and the only thread running is the one executing the
main()function. We can create more threads by calling
pthread_create()and passing the pointer to a callback function (
void* (*cb)(void*);). If we create two threads - one for function
a()and one for function
b()- we will end up with three threads:
- Thread executing a()
- Thread executing b()
- Thread executing main()
Once threads are created they are marked as , the scheduler will grant them the opportunity to execute at some point. The
main()thread, on the other hand, is just going to continue its execution as normal. If the main thread encounters
return 0;the operating system is going to kill the program along with all the threads started. To prevent this from happening, we can use
pthread_join()to tell the main thread to wait for other threads to finish. Since other threads contain infinite loops, they are never going to finish. The to thread joining was putting an infinite loop in the main function just after thread creation. Most pthread functions return 0 upon success and a value smaller than 0 upon error. We can use
assert()as a rudimentary runtime error check.
The complete code:
// main.c #include <stdio.h> #include <pthread.h> #include <assert.h> void* a(void* data) { for ( ; ; ) { printf("a"); } return NULL; } void* b(void* data) { for ( ; ; ) { printf("b"); } return NULL; } int main() { pthread_t ta, tb; assert(pthread_create(&ta, NULL, a, NULL) == 0); assert(pthread_create(&tb, NULL, b, NULL) == 0); assert(pthread_join(ta, NULL) == 0); assert(pthread_join(tb, NULL) == 0); return 0; }
Compiling the code above with
gcc -pthread main.cand running it with
./a.outshould print "aaaabbbbbaaaabbbbb". Since the program will never terminate, it can be interrupted with
Ctrl+Cwhich is going to send
SIGINTto the program which, by default, will make it terminate.
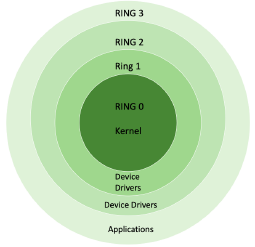
R: 0 / I: 0 (sticky)
This board is for the discussion of operating systems.
- Homework questions must be posted in /hr/ - Homework/Requests
- Tech support belongs to /ts/ - Tech Support
- Testing Mathchan's markup should only be done on /tex/ - LaTeX
- Questions about the board itself can be asked in /meta/ - Meta Questions